Next: volatile , Up: Type Qualifiers [ Contents ][ Index ]

21.1 const Variables and Fields
You can mark a variable as “constant” by writing const in front of the declaration. This says to treat any assignment to that variable as an error. It may also permit some compiler optimizations—for instance, to fetch the value only once to satisfy multiple references to it. The construct looks like this:
After this definition, the code can use the variable pi but cannot assign a different value to it.
Simple variables that are constant can be used for the same purposes as enumeration constants, and they are not limited to integers. The constantness of the variable propagates into pointers, too.
A pointer type can specify that the target is constant. For example, the pointer type const double * stands for a pointer to a constant double . That’s the type that results from taking the address of pi . Such a pointer can’t be dereferenced in the left side of an assignment.
Nonconstant pointers can be converted automatically to constant pointers, but not vice versa. For instance,
This is not an ironclad protection against modifying the value. You can always cast the constant pointer to a nonconstant pointer type:
However, const provides a way to show that a certain function won’t modify the data structure whose address is passed to it. Here’s an example:
Using const char * for the parameter is a way of saying this function never modifies the memory of the string itself.
In calling string_length , you can specify an ordinary char * since that can be converted automatically to const char * .
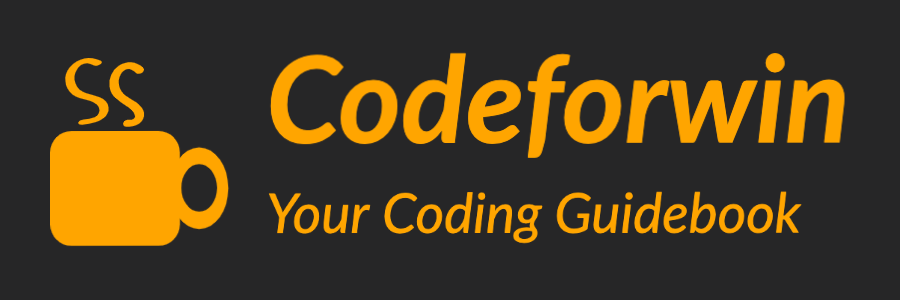
Constant pointer and pointer to constant in C
Quick links.
- Constant pointer
- Pointer to constant
- Constant pointer to constant
Pointers are the most powerful as well as complex component of C programming. For newbies, it’s like learning rocket science in C. However, I have tried my best to simplify things.
In this ongoing series of C programming tutorial, I have explained many concepts related to pointers. Here in this section we will focus on some confusing pointer terminologies. We will learn and compare constant pointer with pointer to constant and constant pointer to constant .
What is constant pointer?
To understand a constant pointer, let us recall definition of a constant variable . Constant variable is a variable whose value cannot be altered throughout the program.
Similarly, constant pointer is a pointer variable whose value cannot be altered throughout the program. It does not allows modification of its value, however you can modify the value pointed by a pointer.
In other words, constant pointer is a pointer that can only point to single object throughout the program.
Syntax to declare constant pointer
Note: You must initialize a constant pointer at the time of its declaration.
Example to declare constant pointer
Note: We use const keyword to declare a constant pointer.
The compiler will generate compilation error on failure of any of the two conditions.
- You must initialize a constant pointer during its declaration.
- A constant pointer must not be re-assigned.
Example program to use constant pointer
Let us write an example program to demonstrate constant pointer in C.
Let us dry run the above program.
- First, we declared two integer variable num1 , num2 and an integer constant pointer const_ptr that points to num1 .
- The statement *const_ptr = 10; assigns 10 to num1 .
- Next we tried re-assignment of constant pointer i.e. const_ptr = &num2; . The statement will generate compilation error, since a constant pointer can only point to single object throughout the program.
- The last two printf() statements are used to test value of num1 .
On compilation it generates following error message.
What is pointer to constant?
Pointer to constant is a pointer that restricts modification of value pointed by the pointer. You can modify pointer value, but you cannot modify the value pointed by pointer.
Note: There is a minor difference between constant pointer and pointer to constant. A constant pointer can only point to single object throughout the program. You can, alter the value pointed by pointer, but cannot alter pointer value. Whereas pointer to a constant cannot modify the value pointed by pointer, but can alter its value.

Syntax to declare pointer to constant
Example to declare pointer to constant, example program to use pointer to constant.
Let us demonstrate pointer to a constant using an example.
The above program generates compilation error, since we tried to assign value to read only memory location (i.e. the value pointed by the pointer). Below is the compilation error generated by *ptr_const = 100;
Note: Pointer to constant restricts modification of value pointed by the pointer. However, do not think that C compiler converts variable pointed by pointer as constant variable.
Pointer to constant does not allows you to modify the pointed value, using pointer. However, you can directly perform modification on variable (without using pointer). For example,
What is constant pointer to constant?
A constant pointer to constant is a combination of constant pointer and pointer to constant . It is a pointer that does not allow modification of pointer value as well as value pointed by the pointer.
Syntax to declare constant pointer to constant
Example to declare constant pointer to constant, example program to use constant pointer to constant.
Let us demonstrate the concept of constant pointer to constant in C program.
The above program generates two compilation error. First in the statement ptr = &num2; since we tried to assign value to a constant pointer. Second in the statement *ptr = 100; since we tried to assign value pointed by a pointer to constant.
Difference between constant pointer, pointer to constant and constant pointer to constant
Okay so got basic picture of a constant pointer, pointer to constant and constant pointer to constant. Let us summarize the things for quick recap.
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
Difference between constant pointer, pointers to constant, and constant pointers to constants
In this article, we will discuss the differences between constant pointer , pointers to constant & constant pointers to constants . Pointers are the variables that hold the address of some other variables, constants, or functions. There are several ways to qualify pointers using const .
- Pointers to constant.
- Constant pointers.
- Constant pointers to constant.
Pointers to constant :
In the pointers to constant, the data pointed by the pointer is constant and cannot be changed. Although, the pointer itself can change and points somewhere else (as the pointer itself is a variable).
Below is the program to illustrate the same:
Constant pointers :
In constant pointers, the pointer points to a fixed memory location , and the value at that location can be changed because it is a variable, but the pointer will always point to the same location because it is made constant here.
Below is an example to understand the constant pointers with respect to references. It can be assumed references as constant pointers which are automatically dereferenced. The value passed in the actual parameter can be changed but the reference points to the same variable.
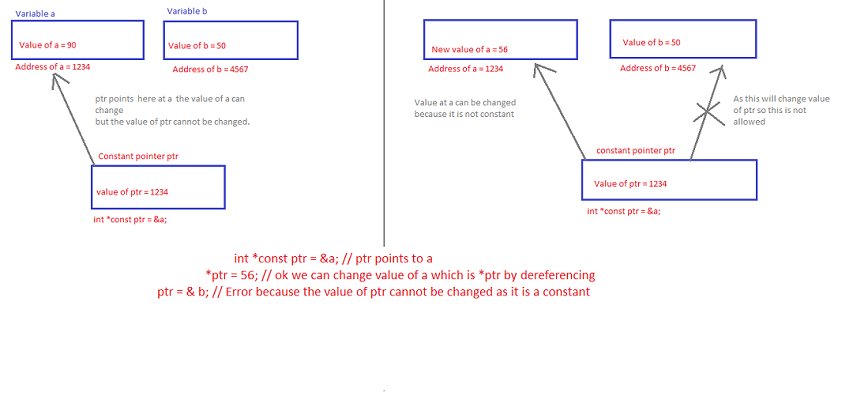
Constant Pointers to constants :
In the constant pointers to constants, the data pointed to by the pointer is constant and cannot be changed. The pointer itself is constant and cannot change and point somewhere else. Below is the image to illustrate the same:
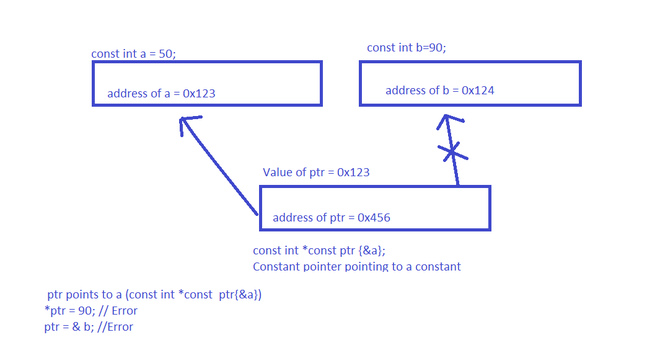
Similar Reads
- Advanced Pointer
- C-Pointer Basics
Please Login to comment...
Improve your coding skills with practice.
What kind of Experience do you want to share?

COMMENTS
A const char* is a pointer to memory that cannot be modified using that pointer. Nothing here can circumvent that. The compiler would object if you assigned c4 = c1 since then …
A pointer type can specify that the target is constant. For example, the pointer type const double * stands for a pointer to a constant double. That’s the type that results from taking the address of …
You must initialize a constant pointer during its declaration. A constant pointer must not be re-assigned. Example program to use constant pointer. Let us write an example …
Pointers in C are variables that are used to store the memory address of another variable. Pointers allow us to efficiently manage the memory and hence optimize our program. In this article, we will discuss some of the …
In this article, we will discuss the differences between constant pointer, pointers to constant & constant pointers to constants. Pointers are the variables that hold the address of some other variables, constants, or …
To declare a const pointer in C, you use the const keyword before the pointer’s data type. For example, `const int *ptr` declares a pointer to an integer that cannot be …